Last Updated on November 15, 2022
PyTorch is an open-source deep studying framework primarily based on Python language. It means that you can construct, prepare, and deploy deep studying fashions, providing quite a lot of versatility and effectivity.
PyTorch is primarily centered on tensor operations whereas a tensor generally is a quantity, matrix, or a multi-dimensional array.
In this tutorial, we are going to carry out some primary operations on one-dimensional tensors as they’re advanced mathematical objects and a necessary a part of the PyTorch library. Therefore, earlier than going into the element and extra superior ideas, one ought to know the fundamentals.
After going by means of this tutorial, you’ll:
- Understand the fundamentals of one-dimensional tensor operations in PyTorch.
- Know about tensor sorts and shapes and carry out tensor slicing and indexing operations.
- Be in a position to apply some strategies on tensor objects, reminiscent of imply, commonplace deviation, addition, multiplication, and extra.
Let’s get began.
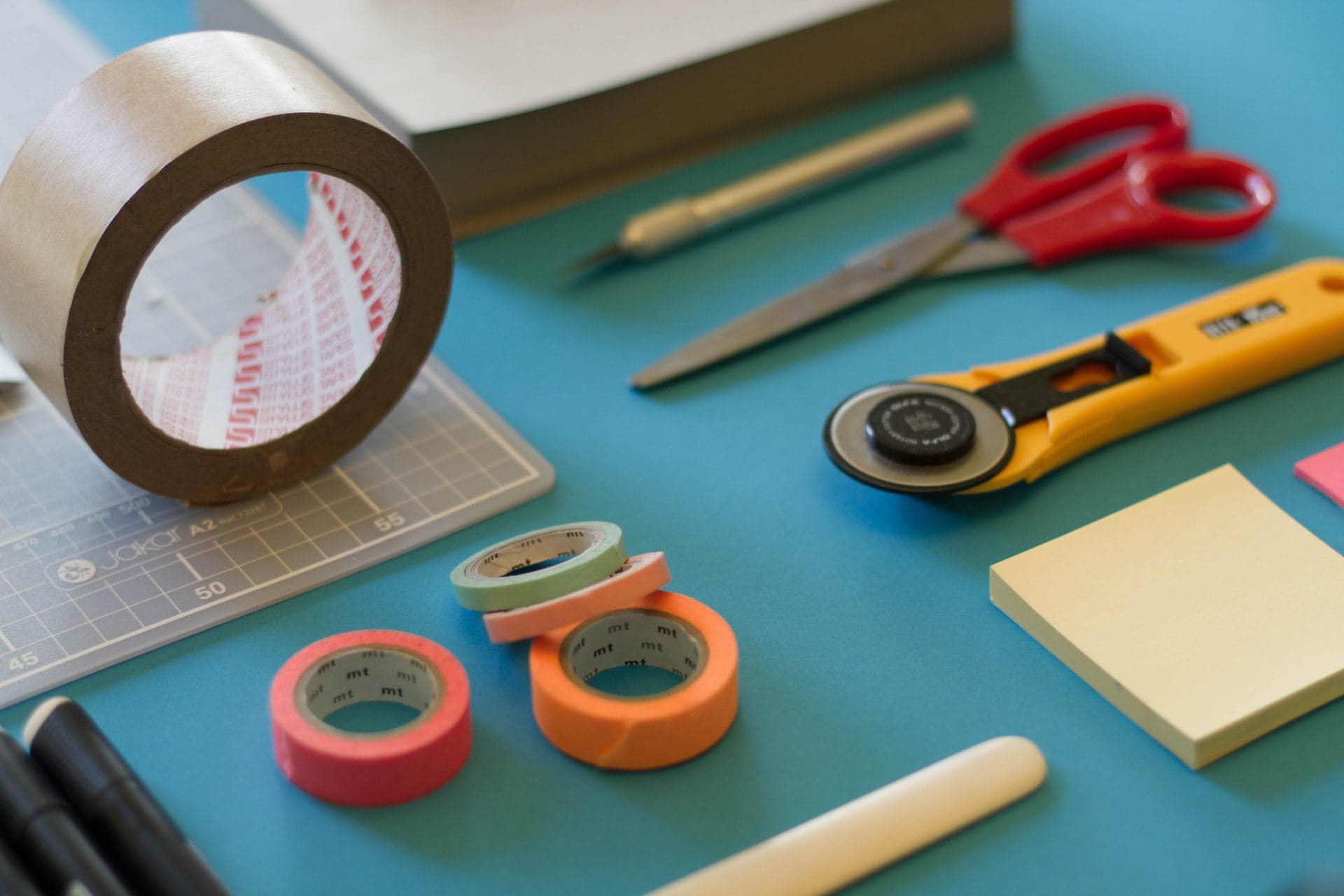
One-Dimensional Tensors in Pytorch
Picture by Jo Szczepanska. Some rights reserved.
Types and Shapes of One-Dimensional Tensors
First off, let’s import a number of libraries we’ll use on this tutorial.
import torch import numpy as np import pandas as pd |
If you may have expertise in different programming languages, the simplest method to perceive a tensor is to contemplate it as a multidimensional array. Therefore, a one-dimensional tensor is solely a one-dimensional array, or a vector. In order to transform an inventory of integers to tensor, apply torch.tensor()
constructor. For occasion, we’ll take an inventory of integers and convert it to numerous tensor objects.
int_to_tensor = torch.tensor([10, 11, 12, 13]) print(“Tensor object kind after conversion: “, int_to_tensor.dtype) print(“Tensor object kind after conversion: “, int_to_tensor.kind()) |
Tensor object kind after conversion: torch.int64 Tensor object kind after conversion: torch.LengthyTensor |
Also, you possibly can apply the identical technique torch.tensor() to transform a float listing to a float tensor.
float_to_tensor = torch.tensor([10.0, 11.0, 12.0, 13.0]) print(“Tensor object kind after conversion: “, float_to_tensor.dtype) print(“Tensor object kind after conversion: “, float_to_tensor.kind()) |
Tensor object kind after conversion: torch.float32 Tensor object kind after conversion: torch.FloatTensor |
Note that parts of an inventory that should be transformed right into a tensor will need to have the identical kind. Moreover, if you wish to convert an inventory to a sure tensor kind, torch additionally means that you can try this. The code traces under, for instance, will convert an inventory of integers to a float tensor.
int_list_to_float_tensor = torch.FloatTensor([10, 11, 12, 13]) int_list_to_float_tensor.kind() print(“Tensor kind after conversion: “, int_list_to_float_tensor.kind()) |
Tensor kind after conversion: torch.FloatTensor |
Similarly, measurement()
and ndimension()
strategies will let you discover the dimensions and dimensions of a tensor object.
print(“Size of the int_list_to_float_tensor: “, int_list_to_float_tensor.measurement()) print(“Dimensions of the int_list_to_float_tensor: “,int_list_to_float_tensor.ndimension()) |
Size of the int_list_to_float_tensor: torch.Size([4]) Dimensions of the int_list_to_float_tensor: 1 |
For reshaping a tensor object, view()
technique may be utilized. It takes rows
and columns
as arguments. As an instance, let’s use this technique to reshape int_list_to_float_tensor
.
reshaped_tensor = int_list_to_float_tensor.view(4, 1) print(“Original Size of the tensor: “, reshaped_tensor) print(“New measurement of the tensor: “, reshaped_tensor) |
Original Size of the tensor: tensor([[10.], [11.], [12.], [13.]]) New measurement of the tensor: tensor([[10.], [11.], [12.], [13.]]) |
As you possibly can see, the view()
technique has modified the dimensions of the tensor to torch.Size([4, 1])
, with 4 rows and 1 column.
While the variety of parts in a tensor object ought to stay fixed after view()
technique is utilized, you should use -1
(reminiscent of reshaped_tensor.view(-1, 1)
) to reshape a dynamic-sized tensor.
Converting Numpy Arrays to Tensors
Pytorch additionally means that you can convert NumPy arrays to tensors. You can use torch.from_numpy
for this operation. Let’s take a NumPy array and apply the operation.
print(“dtype of the tensor: “, from_numpy_to_tensor.dtype)
print(“kind of the tensor: “, from_numpy_to_tensor.kind())
numpy_arr = np.array([10.0, 11.0, 12.0, 13.0]) from_numpy_to_tensor = torch.from_numpy(numpy_arr)
print(“dtype of the tensor: “, from_numpy_to_tensor.dtype) print(“kind of the tensor: “, from_numpy_to_tensor.kind()) |
dtype of the tensor: torch.float64 kind of the tensor: torch.DoubleTensor |
Similarly, you possibly can convert the tensor object again to a NumPy array. Let’s use the earlier instance to point out the way it’s completed.
tensor_to_numpy = from_numpy_to_tensor.numpy() print(“again to numpy from tensor: “, tensor_to_numpy) print(“dtype of transformed numpy array: “, tensor_to_numpy.dtype) |
again to numpy from tensor: [10. 11. 12. 13.] dtype of transformed numpy array: float64 |
Converting Pandas Series to Tensors
You can even convert a pandas sequence to a tensor. For this, first you’ll must retailer the pandas sequence with values()
operate utilizing a NumPy array.
pandas_series=pd.Series([1, 0.2, 3, 13.1]) store_with_numpy=torch.from_numpy(pandas_series.values) print(“Stored tensor in numpy array: “, store_with_numpy) print(“dtype of saved tensor: “, store_with_numpy.dtype) print(“kind of saved tensor: “, store_with_numpy.kind()) |
Stored tensor in numpy array: tensor([ 1.0000, 0.2000, 3.0000, 13.1000], dtype=torch.float64) dtype of saved tensor: torch.float64 kind of saved tensor: torch.DoubleTensor |
Furthermore, the Pytorch framework permits us to do loads with tensors reminiscent of its merchandise()
technique returns a python quantity from a tensor and tolist()
technique returns an inventory.
new_tensor=torch.tensor([10, 11, 12, 13]) print(“the second merchandise is”,new_tensor[1].merchandise()) tensor_to_list=new_tensor.tolist() print(‘tensor:’, new_tensor,“nlist:”,tensor_to_list) |
the second merchandise is 11 tensor: tensor([10, 11, 12, 13]) listing: [10, 11, 12, 13] |
Indexing and Slicing in One-Dimensional Tensors
Indexing and slicing operations are nearly the identical in Pytorch as python. Therefore, the primary index at all times begins at 0 and the final index is lower than the full size of the tensor. Use sq. brackets to entry any quantity in a tensor.
tensor_index = torch.tensor([0, 1, 2, 3]) print(“Check worth at index 0:”,tensor_index[0]) print(“Check worth at index 3:”,tensor_index[3]) |
Check worth at index 0: tensor(0) Check worth at index 3: tensor(3) |
Like an inventory in python, you may as well carry out slicing operations on the values in a tensor. Moreover, the Pytorch library means that you can change sure values in a tensor as nicely.
Let’s take an instance to examine how these operations may be utilized.
example_tensor = torch.tensor([50, 11, 22, 33, 44]) sclicing_tensor = example_tensor[1:4] print(“instance tensor : “, example_tensor) print(“subset of instance tensor:”, sclicing_tensor) |
instance tensor : tensor([50, 11, 22, 33, 44]) subset of instance tensor: tensor([11, 22, 33]) |
Now, let’s change the worth at index 3 of example_tensor
:
print(“worth at index 3 of instance tensor:”, example_tensor[3]) example_tensor[3] = 0 print(“new tensor:”, example_tensor) |
worth at index 3 of instance tensor: tensor(0) new tensor: tensor([50, 11, 22, 0, 44]) |
Some Functions to Apply on One-Dimensional Tensors
In this part, we’ll overview some statistical strategies that may be utilized on tensor objects.
Min and Max Functions
These two helpful strategies are employed to seek out the minimal and most worth in a tensor. Here is how they work.
We’ll use a sample_tensor
for instance to use these strategies.
sample_tensor = torch.tensor([5, 4, 3, 2, 1]) min_value = sample_tensor.min() max_value = sample_tensor.max() print(“examine minimal worth within the tensor: “, min_value) print(“examine most worth within the tensor: “, max_value) |
examine minimal worth within the tensor: tensor(1) examine most worth within the tensor: tensor(5) |
Mean and Standard Deviation
Mean and commonplace deviation are sometimes used whereas doing statistical operations on tensors. You can apply these two metrics utilizing .imply()
and .std()
capabilities in Pytorch.
Let’s use an instance to see how these two metrics are calculated.
mean_std_tensor = torch.tensor([–1.0, 2.0, 1, –2]) Mean = mean_std_tensor.imply() print(“imply of mean_std_tensor: “, Mean) std_dev = mean_std_tensor.std() print(“commonplace deviation of mean_std_tensor: “, std_dev) |
imply of mean_std_tensor: tensor(0.) commonplace deviation of mean_std_tensor: tensor(1.8257) |
Simple Addition and Multiplication Operations on One-Dimensional Tensors
Addition and Multiplication operations may be simply utilized on tensors in Pytorch. In this part, we’ll create two one-dimensional tensors to show how these operations can be utilized.
add = a + b
multiply = a * b
print(“addition of two tensors: “, add)
print(“multiplication of two tensors: “, multiply)
a = torch.tensor([1, 1]) b = torch.tensor([2, 2])
add = a + b multiply = a * b
print(“addition of two tensors: “, add) print(“multiplication of two tensors: “, multiply) |
addition of two tensors: tensor([3, 3]) multiplication of two tensors: tensor([2, 2]) |
For your comfort, under is all of the examples above tying collectively so you possibly can strive them in a single shot:
int_to_tensor = torch.tensor([10, 11, 12, 13])
print(“Tensor object kind after conversion: “, int_to_tensor.dtype)
print(“Tensor object kind after conversion: “, int_to_tensor.kind())
float_to_tensor = torch.tensor([10.0, 11.0, 12.0, 13.0])
print(“Tensor object kind after conversion: “, float_to_tensor.dtype)
print(“Tensor object kind after conversion: “, float_to_tensor.kind())
int_list_to_float_tensor = torch.FloatTensor([10, 11, 12, 13])
int_list_to_float_tensor.kind()
print(“Tensor kind after conversion: “, int_list_to_float_tensor.kind())
print(“Size of the int_list_to_float_tensor: “, int_list_to_float_tensor.measurement())
print(“Dimensions of the int_list_to_float_tensor: “,int_list_to_float_tensor.ndimension())
reshaped_tensor = int_list_to_float_tensor.view(4, 1)
print(“Original Size of the tensor: “, reshaped_tensor)
print(“New measurement of the tensor: “, reshaped_tensor)
numpy_arr = np.array([10.0, 11.0, 12.0, 13.0])
from_numpy_to_tensor = torch.from_numpy(numpy_arr)
print(“dtype of the tensor: “, from_numpy_to_tensor.dtype)
print(“kind of the tensor: “, from_numpy_to_tensor.kind())
tensor_to_numpy = from_numpy_to_tensor.numpy()
print(“again to numpy from tensor: “, tensor_to_numpy)
print(“dtype of transformed numpy array: “, tensor_to_numpy.dtype)
pandas_series=pd.Series([1, 0.2, 3, 13.1])
store_with_numpy=torch.from_numpy(pandas_series.values)
print(“Stored tensor in numpy array: “, store_with_numpy)
print(“dtype of saved tensor: “, store_with_numpy.dtype)
print(“kind of saved tensor: “, store_with_numpy.kind())
new_tensor=torch.tensor([10, 11, 12, 13])
print(“the second merchandise is”,new_tensor[1].merchandise())
tensor_to_list=new_tensor.tolist()
print(‘tensor:’, new_tensor,”nlist:”,tensor_to_list)
tensor_index = torch.tensor([0, 1, 2, 3])
print(“Check worth at index 0:”,tensor_index[0])
print(“Check worth at index 3:”,tensor_index[3])
example_tensor = torch.tensor([50, 11, 22, 33, 44])
sclicing_tensor = example_tensor[1:4]
print(“instance tensor : “, example_tensor)
print(“subset of instance tensor:”, sclicing_tensor)
print(“worth at index 3 of instance tensor:”, example_tensor[3])
example_tensor[3] = 0
print(“new tensor:”, example_tensor)
sample_tensor = torch.tensor([5, 4, 3, 2, 1])
min_value = sample_tensor.min()
max_value = sample_tensor.max()
print(“examine minimal worth within the tensor: “, min_value)
print(“examine most worth within the tensor: “, max_value)
mean_std_tensor = torch.tensor([-1.0, 2.0, 1, -2])
Mean = mean_std_tensor.imply()
print(“imply of mean_std_tensor: “, Mean)
std_dev = mean_std_tensor.std()
print(“commonplace deviation of mean_std_tensor: “, std_dev)
a = torch.tensor([1, 1])
b = torch.tensor([2, 2])
add = a + b
multiply = a * b
print(“addition of two tensors: “, add)
print(“multiplication of two tensors: “, multiply)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 |
import torch import numpy as np import pandas as pd
int_to_tensor = torch.tensor([10, 11, 12, 13]) print(“Tensor object kind after conversion: “, int_to_tensor.dtype) print(“Tensor object kind after conversion: “, int_to_tensor.kind())
float_to_tensor = torch.tensor([10.0, 11.0, 12.0, 13.0]) print(“Tensor object kind after conversion: “, float_to_tensor.dtype) print(“Tensor object kind after conversion: “, float_to_tensor.kind())
int_list_to_float_tensor = torch.FloatTensor([10, 11, 12, 13]) int_list_to_float_tensor.kind() print(“Tensor kind after conversion: “, int_list_to_float_tensor.kind())
print(“Size of the int_list_to_float_tensor: “, int_list_to_float_tensor.measurement()) print(“Dimensions of the int_list_to_float_tensor: “,int_list_to_float_tensor.ndimension())
reshaped_tensor = int_list_to_float_tensor.view(4, 1) print(“Original Size of the tensor: “, reshaped_tensor) print(“New measurement of the tensor: “, reshaped_tensor)
numpy_arr = np.array([10.0, 11.0, 12.0, 13.0]) from_numpy_to_tensor = torch.from_numpy(numpy_arr) print(“dtype of the tensor: “, from_numpy_to_tensor.dtype) print(“kind of the tensor: “, from_numpy_to_tensor.kind())
tensor_to_numpy = from_numpy_to_tensor.numpy() print(“again to numpy from tensor: “, tensor_to_numpy) print(“dtype of transformed numpy array: “, tensor_to_numpy.dtype)
pandas_series=pd.Series([1, 0.2, 3, 13.1]) store_with_numpy=torch.from_numpy(pandas_series.values) print(“Stored tensor in numpy array: “, store_with_numpy) print(“dtype of saved tensor: “, store_with_numpy.dtype) print(“kind of saved tensor: “, store_with_numpy.kind())
new_tensor=torch.tensor([10, 11, 12, 13]) print(“the second merchandise is”,new_tensor[1].merchandise()) tensor_to_list=new_tensor.tolist() print(‘tensor:’, new_tensor,“nlist:”,tensor_to_list)
tensor_index = torch.tensor([0, 1, 2, 3]) print(“Check worth at index 0:”,tensor_index[0]) print(“Check worth at index 3:”,tensor_index[3])
example_tensor = torch.tensor([50, 11, 22, 33, 44]) sclicing_tensor = example_tensor[1:4] print(“instance tensor : “, example_tensor) print(“subset of instance tensor:”, sclicing_tensor)
print(“worth at index 3 of instance tensor:”, example_tensor[3]) example_tensor[3] = 0 print(“new tensor:”, example_tensor)
sample_tensor = torch.tensor([5, 4, 3, 2, 1]) min_value = sample_tensor.min() max_value = sample_tensor.max() print(“examine minimal worth within the tensor: “, min_value) print(“examine most worth within the tensor: “, max_value)
mean_std_tensor = torch.tensor([–1.0, 2.0, 1, –2]) Mean = mean_std_tensor.imply() print(“imply of mean_std_tensor: “, Mean) std_dev = mean_std_tensor.std() print(“commonplace deviation of mean_std_tensor: “, std_dev)
a = torch.tensor([1, 1]) b = torch.tensor([2, 2]) add = a + b multiply = a * b print(“addition of two tensors: “, add) print(“multiplication of two tensors: “, multiply) |
Further Reading
Developed concurrently TensorMovement, PyTorch used to have a less complicated syntax till TensorMovement adopted Keras in its 2.x model. To study the fundamentals of PyTorch, chances are you’ll need to learn the PyTorch tutorials:
Especially the fundamentals of PyTorch tensor may be discovered within the Tensor tutorial web page:
There are additionally fairly a number of books on PyTorch which might be appropriate for novices. A extra just lately revealed ebook must be really useful because the instruments and syntax are actively evolving. One instance is
Summary
In this tutorial, you’ve found learn how to use one-dimensional tensors in Pytorch.
Specifically, you discovered:
- The fundamentals of one-dimensional tensor operations in PyTorch
- About tensor sorts and shapes and learn how to carry out tensor slicing and indexing operations
- How to use some strategies on tensor objects, reminiscent of imply, commonplace deviation, addition, and multiplication