If you’ve been topic to my posts on Twitter or LinkedIn, you will have seen that I’ve accomplished no writing within the final 6 months. Besides the entire… full-time job factor … that is additionally as a result of at first of the yr I made a decision to give attention to a bigger coding challenge.
At my earlier job, I stood up a system for process and movement planning (TAMP) utilizing the Toyota Human Support Robot (HSR). You can study extra in my 2020 recap publish. While I’m actually capable of speak about that work, the code itself was closed in two other ways:
- Research collaborations with Toyota Research Institute (TRI) pertaining to the HSR are in a closed group, except some publicly obtainable repositories constructed across the RoboCup@Home Domestic Standard Platform League (DSPL).
- The code not particular to the robotic itself was contained in a personal repository in my former group’s group, and moreover is embedded in a large monorepo.
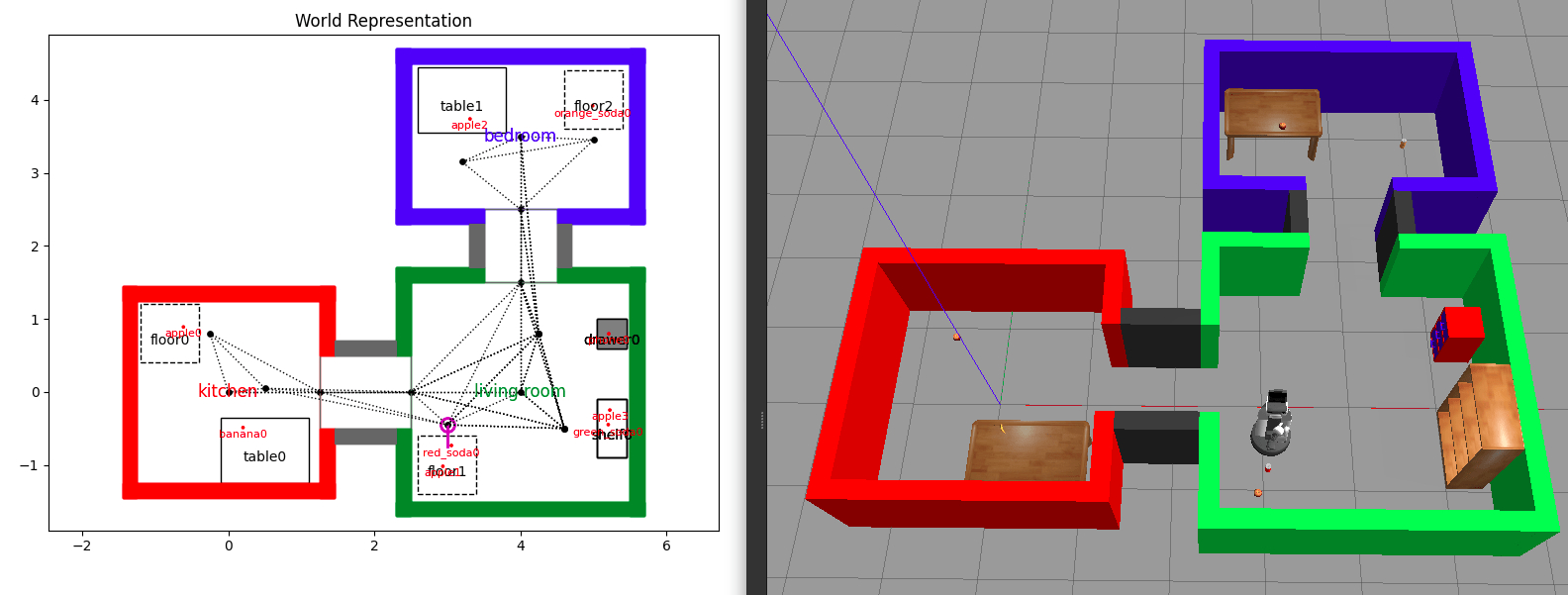
Rewind to 2020: The authentic simulation device (left) and a generated Gazebo world with a Toyota HSR (proper).
So I believed, there are some generic utilities right here that might be helpful to the group. What wouldn’t it take to strip out the house service robotics simulation instruments out of that setting and make it obtainable as a standalone bundle? Also, how might I squeeze in enhancements and study attention-grabbing issues alongside the way in which?
This publish describes how these utilities grew to become pyrobosim: A ROS2 enabled 2D cell robotic simulator for conduct prototyping.
What is pyrobosim?
At its core, pyrobosim is a straightforward robotic conduct simulator tailor-made for family environments, however helpful to different purposes with comparable assumptions: transferring, selecting, and putting objects in a 2.5D* world.
* For these unfamiliar, 2.5D usually describes a 2D surroundings with restricted entry to a 3rd dimension. In the case of pyrobosim, this implies all navigation occurs in a 2D aircraft, however manipulation duties happen at a selected top above the bottom aircraft.
The supposed workflow is:
- Use pyrobosim to construct a world and prototype your conduct
- Generate a Gazebo world and run with a higher-fidelity robotic mannequin
- Run on the true robotic!
Pyrobosim lets you outline worlds made up of entities. These are:
- Robots: Programmable brokers that may act on the world to alter its state.
- Rooms: Polygonal areas that the robotic can navigate, related by Hallways.
- Locations: Polygonal areas that the robotic can’t drive into, however could comprise manipulable objects. Locations comprise one in every of extra Object Spawns. This permits having a number of object spawns in a single entity (for instance, a left and proper countertop).
- Objects: The issues that the robotic can transfer to alter the state of the world.
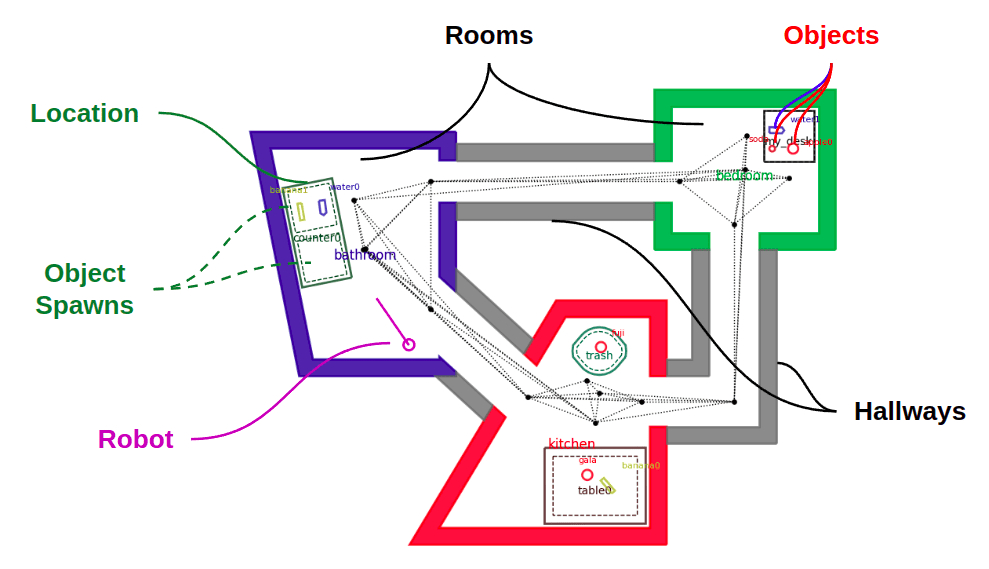
Main entity varieties proven in a pyrobosim world.
Given a static set of rooms, hallways, and areas, a robotic on the earth can then take actions to alter the state of the world. The principal 3 actions applied are:
- Pick: Remove an object from a location and maintain it.
- Place: Put a held object at a selected location and pose inside that location.
- Navigate: Plan and execute a path to maneuver the robotic from one pose to a different.
As that is primarily a cell robotic simulator, there may be extra give attention to navigation vs. manipulation options. While selecting and putting are idealized, which is why we are able to get away with a 2.5D world illustration, the thought is that the trail planners and path followers might be swapped out to check totally different navigation capabilities.
Another long-term imaginative and prescient for this device is that the set of actions itself might be expanded. Some random concepts embody transferring furnishings, opening and shutting doorways, or gaining data in partially observable worlds (for instance, an express “scan” motion).
Independently of the checklist of potential actions and their parameters, these actions can then be sequenced right into a plan. This plan might be manually specified (“go to A”, “pick up B”, and so on.) or the output of a higher-level process planner which takes in a process specification and outputs a plan that satisfies the specification.

Execution of a pattern motion sequence in pyrobosim.
In abstract: pyrobosim is a software program device the place you’ll be able to transfer an idealized level robotic round a world, choose and place objects, and check process and movement planners earlier than transferring into higher-fidelity settings — whether or not it’s different simulators or an actual robotic.
What’s new?
Taking this code out of its authentic resting spot was removed from a copy-paste train. While sifting via the code, I made a couple of enhancements and design adjustments with modularity in thoughts: ROS vs. no ROS, GUI vs. no GUI, world vs. robotic capabilities, and so forth. I additionally added new options with the egocentric agenda of studying issues I needed to attempt… which is the purpose of a enjoyable private aspect challenge, proper?
Let’s dive into a couple of key thrusts that made up this preliminary launch of pyrobosim.
1. User expertise
The authentic device was carefully tied to a single Matplotlib determine window that had to be open, and usually there have been a number of shortcuts to simply get the factor to work. In this redesign, I attempted to extra cleanly separate the modeling from the visualization, and properties of the world itself with properties of the robotic agent and the actions it might probably take on the earth.
I additionally needed to make the GUI itself a bit nicer. After some fast looking out, I discovered this publish that confirmed tips on how to put a Matplotlib canvas in a PyQT5 GUI, that’s what I went for. For now, I began by including a couple of buttons and edit packing containers that enable interplay with the world. You can write down (or generate) a location identify, see how the present path planner and follower work, and choose and place objects when arriving at particular areas.
In tinkering with this new GUI, I discovered numerous bugs with the unique code which resulted in good basic adjustments within the modeling framework. Or, to make it sound fancier, the GUI offered an important platform for interactive testing.
The last item I did by way of usability was present customers the choice of making worlds with out even touching the Python API. Since the libraries of potential areas and objects had been already outlined in YAML, I threw within the potential to creator the world itself in YAML as effectively. So, in idea, you might take one of many canned demo scripts and swap out the paths to three recordsdata (areas, objects, and world) to have a very totally different instance able to go.
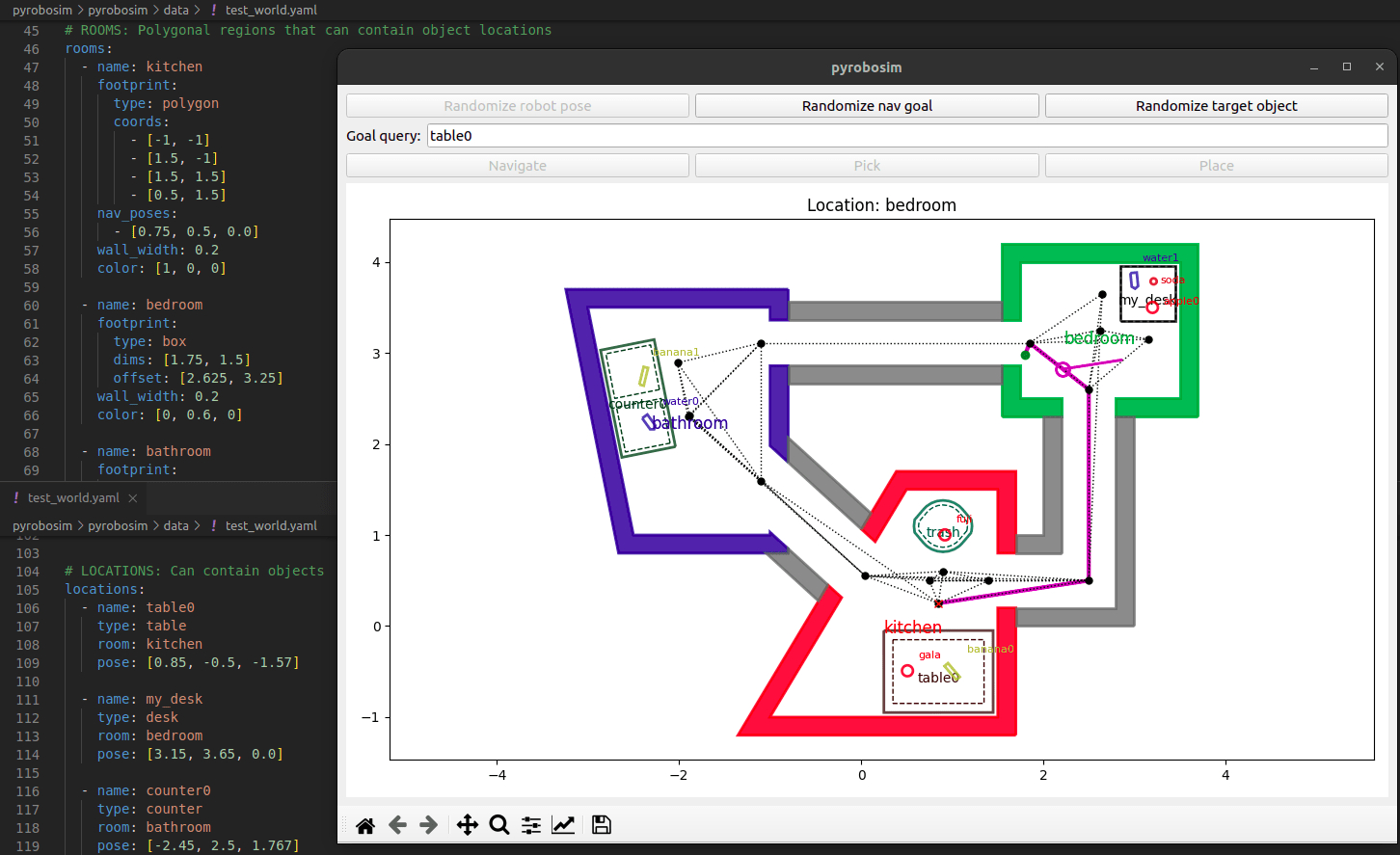
pyrobosim GUI with snippets of the world YAML file behind it.
2. Generalizing movement planning
In the unique device, navigation was so simple as potential as I used to be targeted on actual robotic experiments. All I wanted within the simulated world was a consultant price perform for planning that may approximate how far a robotic must journey from level A to level B.
This resulted in build up a roadmap of (recognized and manually specified) navigation poses round areas and on the heart of rooms and hallways. Once you’ve got this graph illustration of the world, you should use a normal shortest-path search algorithm like A* to discover a path between any two factors in house.
This time round, I needed somewhat extra generality. The design has now advanced to incorporate two standard classes of movement planners.
- Single-query planners: Plans as soon as from the present state of the robotic to a selected objective pose. An instance is the ever-present Rapidly-expanding Random Tree (RRT). Since every robotic plans from its present state, single-query planners are thought-about to be properties of a person robotic in pyrobosim.
- Multi-query planners: Builds a illustration for planning which might be reused for various begin/objective configurations given the world doesn’t change. The authentic hard-coded roadmap suits this invoice, in addition to the sampling-based Probabilistic Roadmap (PRM). Since a number of robots might reuse these planners by connecting begin and objective poses to an present graph, multi-query planners are thought-about properties of the world itself in pyrobosim.
I additionally needed to contemplate path following algorithms sooner or later. For now, the piping is there for robots to swap out totally different path followers, however the one implementation is a “straight line executor”. This assumes the robotic is some extent that may transfer in ultimate straight-line trajectories. Later on, I want to contemplate nonholonomic constraints and allow dynamically possible planning, in addition to true path following which units the rate of the robotic inside some limits quite than teleporting the robotic to ideally comply with a given path.
In common, there are many alternatives so as to add extra of the low-level robotic dynamics to pyrobosim, whereas proper now the main target is basically on the higher-level conduct aspect. Something just like the MATLAB based mostly Mobile Robotics Simulation Toolbox, which I labored on in a former job, has extra of this in place, so it’s actually potential!
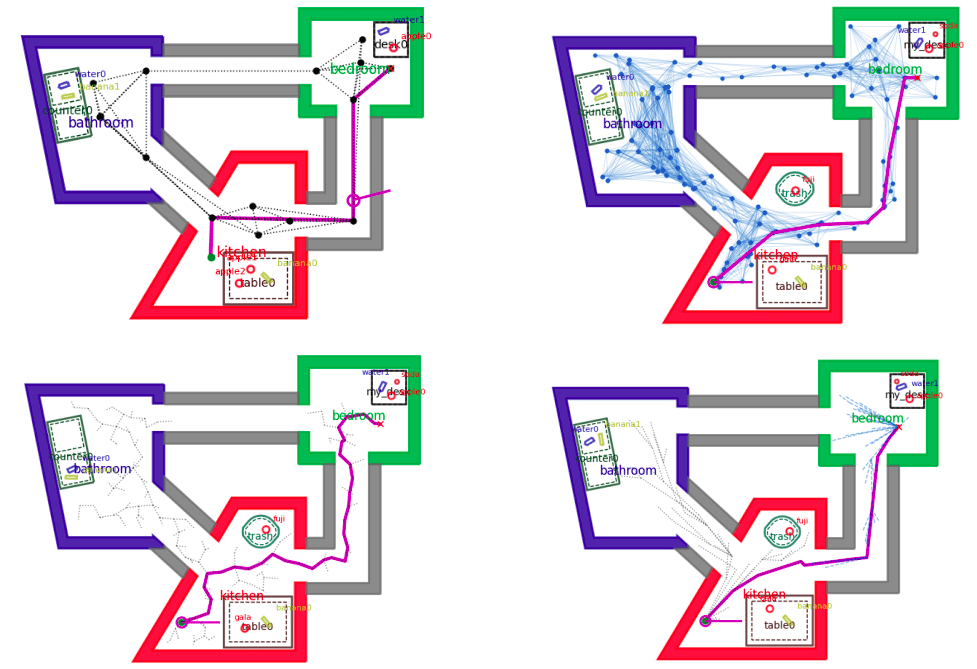
Sample path planners in pyrobosim.
Hard-coded roadmap (higher left), Probabilistic Roadmap (PRM) (higher proper).
Rapidly-expanding Random Tree (RRT) (decrease left), Bidirectional RRT* (decrease proper).
3. Plugging into the most recent ecosystem
This was most likely essentially the most egocentric and pointless replace to the instruments. I needed to play with ROS2, so I made this right into a ROS2 bundle. Simple as that. However, I throttled again on the selfishness sufficient to make sure that every part may be run standalone. In different phrases, I don’t need to require anybody to make use of ROS in the event that they don’t need to.
The ROS method does present a couple of advantages, although:
- Distributed execution: Running the world mannequin, GUI, movement planners, and so on. in a single course of will not be nice, and in reality I bumped into numerous snags with multithreading earlier than I launched ROS into the combo and will cut up items into separate nodes.
- Multi-language interplay: ROS usually is sweet as a result of you’ll be able to have for instance Python nodes interacting with C++ nodes “for free”. I’m particularly excited for this to result in collaborations with attention-grabbing robotics instruments out within the wild.
The different factor that got here with this was the Gazebo world exporting, which was already obtainable within the former code. However, there may be now a more recent Ignition Gazebo and I needed to attempt that as effectively. After discovering that polyline geometries (a key function I relied on) was not supported in Ignition, I complained simply loudly sufficient on Twitter that the lead developer of Gazebo personally let me know when she merged that PR! I used to be so excited that I put in the most recent model of Ignition from supply shortly after and with a couple of tweaks to the mannequin era we now assist each Gazebo traditional and Ignition.
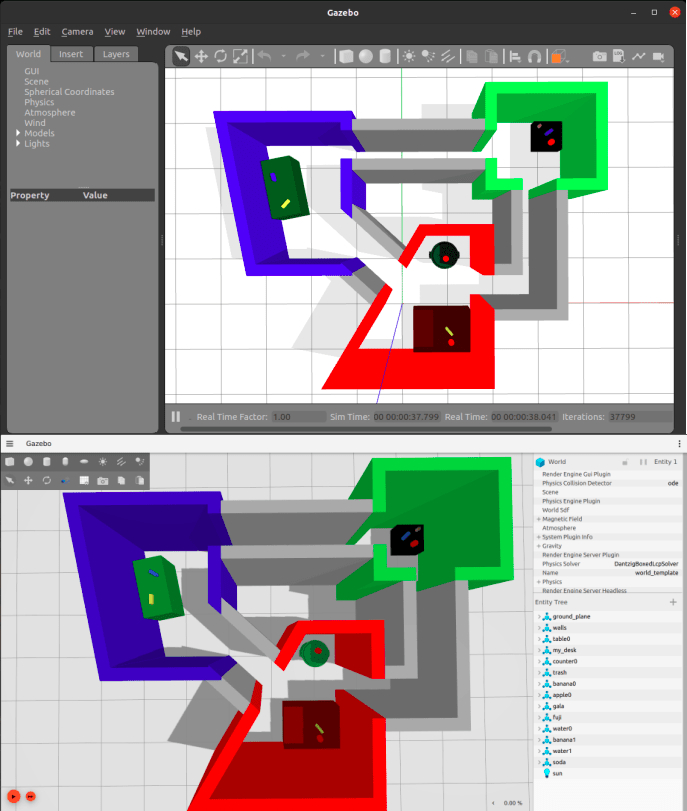
pyrobosim check world exported to Gazebo traditional (prime) and Ignition Gazebo (backside).
4. Software high quality
Some different issues I’ve been eager to attempt for some time relate to good software program improvement practices. I’m joyful that in mentioning pyrobosim, I’ve to this point been capable of arrange a fundamental Continuous Integration / Continuous Development (CI/CD) pipeline and official documentation!
For CI/CD, I made a decision to check out GitHub Actions as a result of they’re tightly built-in with GitHub — and critically, compute is free for public repositories! I had previous expertise organising Jenkins (see my earlier publish), and I’ve to say that GitHub Actions was a lot simpler for this “hobbyist” workflow since I didn’t have to determine the place and tips on how to host the CI server itself.
Documentation was one other factor I used to be deliberate about on this redesign. I used to be at all times impressed after I went into some open-source bundle and located professional-looking documentation with examples, tutorials, and a full API reference. So I seemed round and converged on Sphinx which generates the HTML documentation, and comes with an autodoc module that may robotically convert Python docstrings to an API reference. I then used LearnTheDocs which hosts the documentation on-line (once more, without spending a dime) and robotically rebuilds it once you push to your GitHub repository. The remaining consequence was this pyrobosim documentation web page.
The end result could be very satisfying, although I need to admit that my unit exams are… missing in the intervening time. However, it must be tremendous straightforward so as to add new exams into the present CI/CD pipeline now that every one the infrastructure is in place! And so, the technical debt continues build up.
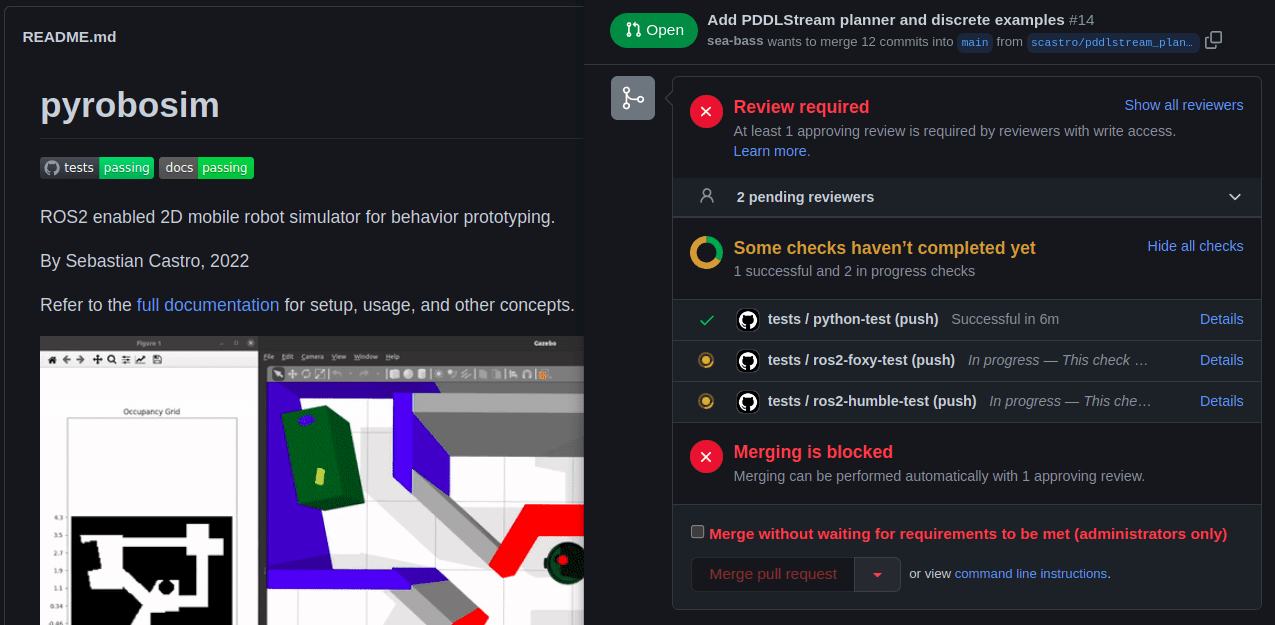
pyrobosim GitHub repo with fairly standing badges (left) and automatic checks in a pull request (proper).
Conclusion / Next steps
This has been an introduction to pyrobosim — each its design philosophy, and the important thing function units I labored on to take the code out of its authentic kind and right into a standalone bundle (hopefully?) worthy of public utilization. For extra data, check out the GitHub repository and the official documentation.
Here is my quick checklist of future concepts, which is on no account full:
- Improving the present instruments: Adding extra unit exams, examples, documentation, and customarily something that makes the pyrobosim a greater expertise for builders and customers alike.
- Building up the navigation stack: I’m significantly all for dynamically possible planners for nonholonomic autos. There are a number of nice instruments on the market to drag from, akin to Peter Corke’s Robotics Toolbox for Python and Atsushi Sakai’s PythonRobotics.
- Adding a conduct layer: Right now, a plan consists of a easy sequence of actions. It’s not very reactive or modular. This is the place abstractions akin to finite-state machines and conduct bushes can be nice to herald.
- Expanding to multi-agent and/or partially-observable methods: Two attention-grabbing instructions that may require main function improvement.
- Collaborating with the group!
It can be implausible to work with a few of you on pyrobosim. Whether you’ve got suggestions on the design itself, particular bug studies, or the flexibility to develop new examples or options, I might admire any type of enter. If you find yourself utilizing pyrobosim in your work, I might be thrilled so as to add your challenge to the checklist of utilization examples!
Finally: I’m at present within the strategy of organising process and movement planning with pyrobosim. Stay tuned for that follow-on publish, which may have a number of cool examples.
You can learn the unique article at Roboticseabass.com.
Sebastian Castro
is a Senior Robotics Engineer at PickNik.